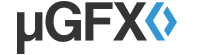 |
µGFX
2.9
version 2.9
|
17 #include "../../../gfx.h"
23 #if !GFX_USE_GDISP || !GDISP_NEED_TEXT
28 #define MF_NO_STDINT_H
32 #define MF_ENCODING MF_ENCODING_UTF8
34 #define MF_ENCODING MF_ENCODING_ASCII
37 #if GDISP_NEED_TEXT_WORDWRAP
38 #define MF_USE_ADVANCED_WORDWRAP 1
40 #define MF_USE_ADVANCED_WORDWRAP 0
43 #define MF_USE_KERNING GDISP_NEED_TEXT_KERNING
44 #define MF_FONT_FILE_NAME "src/gdisp/fonts/fonts.h"
47 #define MF_USE_JUSTIFY 0
54 #ifndef MF_FONT_FILE_NAME
55 #define MF_FONT_FILE_NAME "fonts.h"
72 #define MF_ENCODING_ASCII 0
73 #define MF_ENCODING_UTF8 1
74 #define MF_ENCODING_UTF16 2
75 #define MF_ENCODING_WCHAR 3
77 #define MF_ENCODING MF_ENCODING_UTF8
89 #ifndef MF_KERNING_SPACE_PERCENT
90 #define MF_KERNING_SPACE_PERCENT 15
97 #ifndef MF_KERNING_SPACE_PIXELS
98 #define MF_KERNING_SPACE_PIXELS 3
104 #ifndef MF_KERNING_LIMIT
105 #define MF_KERNING_LIMIT 20
124 #ifndef MF_USE_KERNING
125 #define MF_USE_KERNING 1
131 #ifndef MF_USE_ADVANCED_WORDWRAP
132 #define MF_USE_ADVANCED_WORDWRAP 1
138 #ifndef MF_USE_JUSTIFY
139 #define MF_USE_JUSTIFY 1
146 #define MF_USE_ALIGN 1
153 #define MF_USE_TABS 1
161 #ifndef MF_KERNING_ZONES
162 #define MF_KERNING_ZONES 16
169 #define MF_EXTERN extern "C"