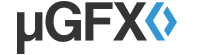 |
µGFX
2.9
version 2.9
|
Go to the documentation of this file.
13 #ifndef _KEYBOARD_MICROCODE_H
14 #define _KEYBOARD_MICROCODE_H
53 #define KMC_HEADERSTART 0x00
54 #define KMC_HEADER_ID1 'L'
55 #define KMC_HEADER_ID2 'M'
56 #define KMC_HEADER_VER_1 0x01
58 #define KMC_HEADER_VER_CURRENT KMC_HEADER_VER_1
59 #define KMC_HEADER_VER_MIN KMC_HEADER_VER_1
60 #define KMC_HEADER_VER_MAX KMC_HEADER_VER_1
62 #define KMC_RECORDSTART 0x01
64 #define KMC_TEST_INIT 0x10
65 #define KMC_TEST_ERROR 0x11
66 #define KMC_TEST_CODE 0x12
67 #define KMC_TEST_CODERANGE 0x13
68 #define KMC_TEST_CODETABLE 0x14
69 #define KMC_TEST_STATEBIT 0x15
70 #define KMC_TEST_STATEOR 0x16
71 #define KMC_TEST_STATEAND 0x17
72 #define KMC_TEST_LAYOUTBIT 0x18
73 #define KMC_TEST_LAYOUTOR 0x19
74 #define KMC_TEST_LAYOUTAND 0x1A
75 #define KMC_TEST_CODEBIT 0x1B
76 #define KMC_TEST_CODEOR 0x1C
77 #define KMC_TEST_CODEAND 0x1D
78 #define KMC_TEST_LASTCODE 0x1E
79 #define KMC_TEST_SHIFT 0x20
80 #define KMC_TEST_NOSHIFT 0x21
81 #define KMC_TEST_CTRL 0x22
82 #define KMC_TEST_NOCTRL 0x23
83 #define KMC_TEST_ALT 0x24
84 #define KMC_TEST_NOALT 0x25
85 #define KMC_TEST_CAPS 0x26
86 #define KMC_TEST_NOCAPS 0x27
87 #define KMC_TEST_NUMLOCK 0x28
88 #define KMC_TEST_NONUMLOCK 0x29
90 #define KMC_ACT_STOP 0xFF
91 #define KMC_ACT_DONE 0xFE
92 #define KMC_ACT_RESET 0xFD
93 #define KMC_ACT_STATEBIT 0x80
94 #define KMC_ACT_LAYOUTBIT 0x81
95 #define KMC_ACT_CODEBIT 0x82
96 #define KMC_ACT_CHAR 0x83
97 #define KMC_ACT_CHARCODE 0x84
98 #define KMC_ACT_CHARRANGE 0x85
99 #define KMC_ACT_CHARTABLE 0x86
100 #define KMC_ACT_CLEAR 0x87
101 #define KMC_ACT_CHARADD 0x88
102 #define KMC_ACT_DATA 0x89
104 #define KMC_BIT_CLEAR 0x80
105 #define KMC_BIT_INVERT 0x40